Leetcode 257 Binary Tree Paths
Given the root of a binary tree, return all root-to-leaf paths in any order.
A leaf is a node with no children.
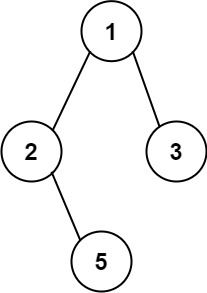
Input: root = [1,2,3,null,5]
Output: ["1->2->5","1->3"]
Input: root = [1]
Output: ["1"]
- Soruda bize bir binary tree veriliyor ve bizden bu ağacın sağ ve sol kollarındaki dalları yazmamız isteniyor.
- DFS kullanarak bu soruyu çözebiliriz.
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, val=0, left=None, right=None):
# self.val = val
# self.left = left
# self.right = right
class Solution:
def binaryTreePaths(self, root: TreeNode) -> List[str]:
output = []
def recursive(cur, res):
if cur.left is None and cur.right is None:
output.append('->'.join(res + [str(cur.val)]))
if cur.left:
recursive(cur.left, res + [str(cur.val)])
if cur.right:
recursive(cur.right, res + [str(cur.val)])
recursive(root, [])
return output