Leetcode 617 Merge Two Binary Trees
You are given two binary trees root1 and root2.
Imagine that when you put one of them to cover the other, some nodes of the two trees are overlapped while the others are not. You need to merge the two trees into a new binary tree. The merge rule is that if two nodes overlap, then sum node values up as the new value of the merged node. Otherwise, the NOT null node will be used as the node of the new tree.
Return the merged tree.
Note: The merging process must start from the root nodes of both trees.
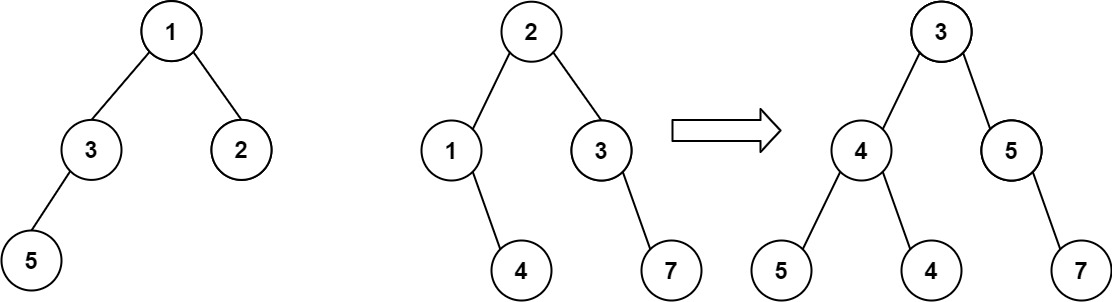
Input: root1 = [1,3,2,5], root2 = [2,1,3,null,4,null,7]
Output: [3,4,5,5,4,null,7]
Input: root1 = [1], root2 = [1,2]
Output: [2,2]
- Bu soruda bizden 2 tane ağacı birleştirmemiz isteniyor.Aslında demek istenen bir ağacı diğerinin üzerine yerleştirmek.Aynı node a denk gelen değerler toplanacak.Eğer o node üzerinde sadece 1 ağacın değeri varsa yeni ağaca da o yerleşecek.
- Burada rekürsif yaklaşım kullanarak her iki ağaçtaki tüm nodeları gezeriz.
- Bir node için her iki ağaçta da değer var ise toplanır.
- Sadece bir tanesinde değer var ise o değer yerleştirilir.
- Daha sonra node’un sağ ve sol child’ları için fonksiyon rekürsif olarak çağrılır.
def mergeTrees(self, root1: TreeNode, root2: TreeNode) -> TreeNode:
if root1 is None:
return root2
if root2 is None:
return root1
root1.val += root2.val
root1.left = self.mergeTrees(root1.left,root2.left)
root1.right = self.mergeTrees(root1.right,root2.right)
return root1